Let’s follow the traders who could gain the highest amount of profit through some online trades in online trading markets. You will gain the best fond ever if you rely on the Aron Group’s copy trading schedule, and there are promises to heal the possible losses during the recent trades. It is wise not to miss the opportunity if you are affected, and don’t let the particular offer end without offering you some compensation.
How does Aron Groups trying to do help the Copy Traders?
Now, Aron Groups is aiming to make Copy Trading easier than ever. Aron Groups has become one of the largest digital copy trading platforms in scale and reliability thanks to its user-friendly design, transparency, and ‘win-win’ trading environment between traders and followers.
Traders can easily choose an expert trader to follow, copy their trading moves, and make profits. When an expert trader opens or closes a trade, the trader then automatically copies that trader without the need for any manual intervention.
Honesty is the foundation of a true partnership. Data is publicly available to ensure a transparent trading environment, and records have been strictly reviewed. The data reflects actual trading behavior on the platform. This way, followers can do their Copy Trading activity safely because all transactions and followers’ records can be monitored on the platform.
Additionally, Aron Groups allows followers to follow multiple traders simultaneously to gain maximum profits. Profit is displayed on tracked trades in real time. Followers can also revise the copy amount and copy position or stop copy orders and close positions to stop loss.
Aron Groups is ready to partly compensate for losses if you can’t find all the mentioned privileges enough. Take advantage of this opportunity because there is a deadline to use the program, just 2-5 October. But how?
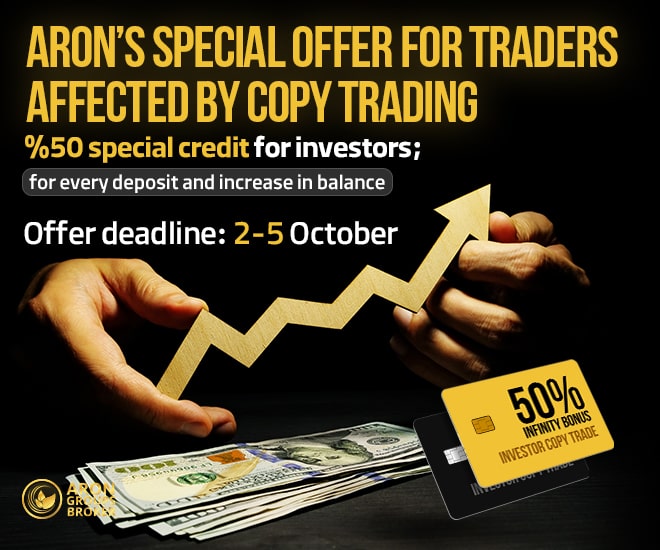
Could I participate the new privilege offered by Aron Groups?
Of course, every trader who has lost some of their capital through Copy Trading activities is welcomed through the promises of this campaign. Just be careful of the following regulations:
• A 50% deposit bonus of up to $750 will be offered to all Aron Groups’ traders who have an Investor Copy Trade account.
• The project starts from 02/10/2023 at 00:01 until 05/10/2023 at 23:59 Country time.
• Acceptable accounts in this plan are Investor Copy Trade ones.
• The minimum deposit amount to take advantage of this program is 100 dollars or its equivalent amount in tomans.
• This bonus will be added to the trader’s Copy Trade account which is considered as a credit that can encounter losses.
• Note that transfers from other trading accounts will not be included in this offer.
• Note that this bonus cannot be withdrawn as credit and can only be counted as your trading account balance.
• This credit is only valid for 30 days from the time of deposit by the broker and will be deleted after the end of the stated period.
• If the stop-out level is reached, the credit will be removed automatically.
• If any transfer or withdrawal happens throughout the trading account to which the credit has been deposited, this gift will be automatically removed.
• The limit of the credit is up to 750 dollars or its equivalent in tomans for bonus.
• Aron Group has the right to deal with the violator in accordance with the rules if they observe any violation or abuse of the rules of the broker. These principles include blocking the account balance, determining and receiving fines or damages, exclusion from broker services, and reckoning.